Creating a Simple “Guess the Number” Game in Python : Pythonista
by: Pythonista
blow post content copied from Finxter
click here to view original post
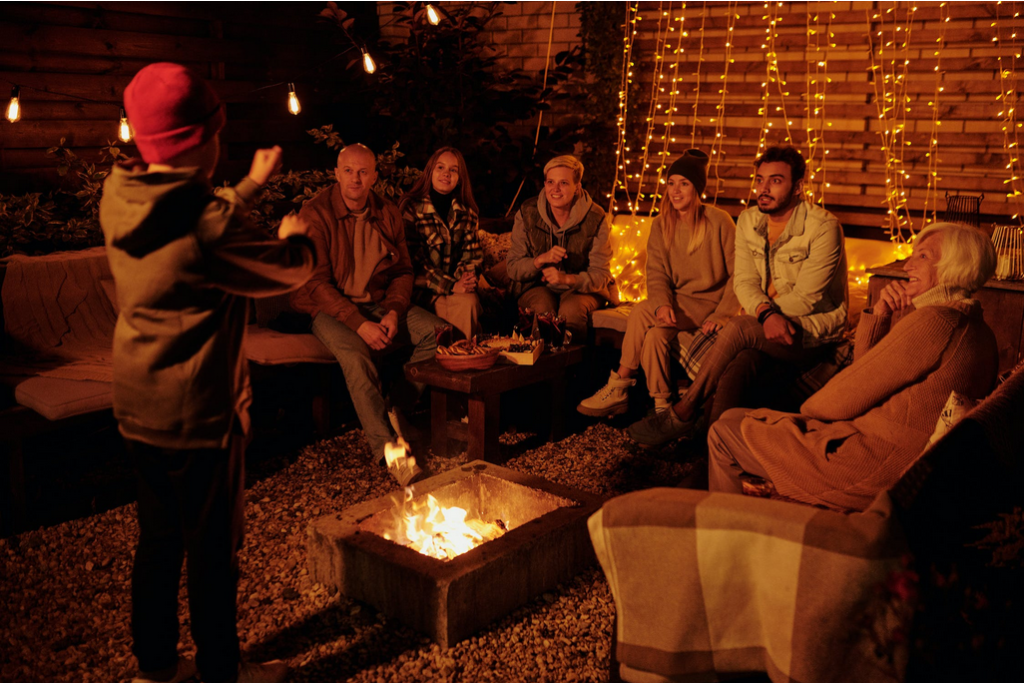
In today’s quick session, I’ll show you a simple Python game that I found interesting because it helps you understand some simple Python concepts such as string formatting and user interaction.
The Game: The Python script will choose a random number between 1 and 10. You attempt to guess the number. The script gives you feedback whether your guess was too high, too low, or correct. It shows the number of trials when you’ve found the magic number!
Visualization Test Run
Here are two test runs of the game, so you know how it works:

Python Code to Implement “Guess The Number” Game
I’ll give you the code right away and follow up with some explanations.
import random max_number = 10 guesses_allowed = 5 print("Welcome to the guessing game!") print("I'm thinking of a number between 1 and {}. Can you guess what it is?".format(max_number)) print(f"You have {} tries to do so.".format(guesses_allowed)) number = random.randint(1, max_number) for i in range(guesses_allowed): guess = int(input("What's your guess? ")) if guess == number: print("Congratulations, you guessed the number in {} tries!".format(i + 1)) break else: if guess > number: print("Your guess is too high. Try again.") else: print("Your guess is too low. Try again.") if guess != number: print("Sorry, you didn't guess the number in {} tries. The game is over.".format(guesses_allowed))
Let’s dive into the explanation of the code:
Python Code Explanation
The code starts by importing the random
module, which is used to generate a random number. The random
module does not have to be installed because it’s already part of Python’s standard library. So it’ll work with any Python installation!
Recommended Tutorial: How to Install Python?
Then, two global variables are defined:
max_number
, which is the maximum number that can be generated by the Python script. This is included, somax_number
can be the “magic number” itself.guesses_allowed
, which is the number of guesses that the player is allowed to make. We set the maximum number of guesses to 5, so the player must find the number in 5 tries.
Next, the game is introduced to the player with a brief message explaining the game:
Welcome to the guessing game!
I'm thinking of a number between 1 and 10. Can you guess what it is?
You have 5 tries to do so.
We use Python’s powerful string formatting capabilities to customize the message to the global variable’s values.
Recommended Tutorial: Python String Formatting
string.format()
Method
Then, a random number is generated using the random.randint()
function, and the game begins.
The game is implemented using a for
loop that runs for guesses_allowed
iterations. On each iteration, the player is prompted to enter their guess using the input()
function. This guess is then compared to the randomly generated number.
If the player’s guess is correct, the game ends and a congratulatory message is displayed. Otherwise, the player is told whether their guess is too high or too low, and the loop continues.
After the for
loop ends, the code checks whether the player has successfully guessed the number. If not, the game is over, and a message is displayed to that effect.
December 11, 2022 at 08:25PM
Click here for more details...
=============================
The original post is available in Finxter by Pythonista
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
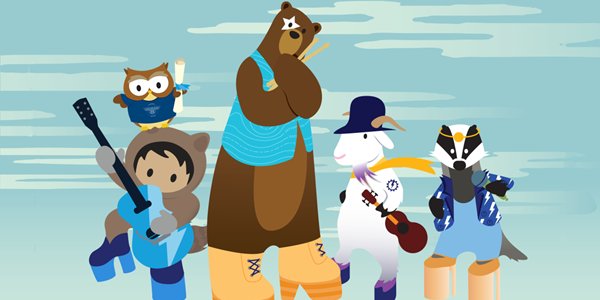
Post a Comment