Level Up Your Python With These 38 Clever One-Liners : Sensei
by: Sensei
blow post content copied from Finxter
click here to view original post
Learning Python one-liners can help you become a more efficient and effective programmer. One-liners enable you to write concise code that can be used to quickly solve problems or automate processes.
Being able to write one-liners can save you time, allow you to quickly debug code, and provide a more succinct way of expressing ideas.
Additionally, having a good understanding of Python one-liners can help you in job interviews and technical assessments.
This article provides you a list of 50 Python One-Liners to learn and improve your skills!
But before we move on, I’m excited to present you my new Python book Python One-Liners (Amazon Link).
If you like one-liners, you’ll LOVE the book. It’ll teach you everything there is to know about a single line of Python code. But it’s also an introduction to computer science, data science, machine learning, and algorithms. The universe in a single line of Python!
The book was released in 2020 with the world-class programming book publisher NoStarch Press (San Francisco).
Link: https://nostarch.com/pythononeliners
One-Liner 1: Generate a Range of Numbers in Python Using a For Loop

This code will print out the numbers 0-9. The for
loop will iterate through the range from 0 to 9, and for each iteration, the number will be printed out.
[print(i) for i in range(10)]
Output:
0
1
2
3
4
5
6
7
8
9
One-Liner 2: Printing Result of Lambda Function Multiplying 2 Numbers
This code is a lambda expression. It is an anonymous function that takes two parameters (a
and b
) and returns the product of a
and b
. In this case, it multiplies 21 and 2 and prints the result, 42.
print((lambda a, b : a * b)(21, 2))
Output:
42
One-Liner 3: Generate a List of Decrementing Integers with Lambda Function
This code will generate a list of numbers where each number is 10 minus the original number. This is achieved using the map
and lambda
functions. The map
function takes the lambda
function and applies it to the range(10)
list, which is 0 to 9. The lambda
function subtracts the number from 10, and the resulting list is printed.
print(list(map(lambda x: 10-x, range(10))))
Output:
[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
One-Liner 4: Generating a Dictionary of Squares from 0 to 9
This code prints a dictionary of key-value pairs, where the keys are the numbers from 0 to 9 and the values are the squares of those numbers. The range()
function creates a list of numbers from 0 to 9, and the dictionary comprehension then creates a dictionary with a key-value pair for each number, where the key is the number and the value is the square of that number.
print({i: i**2 for i in range(10)})
Output:
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
One-Liner 5: Sum of Integers from 0 to 9 Calculated with Python Code
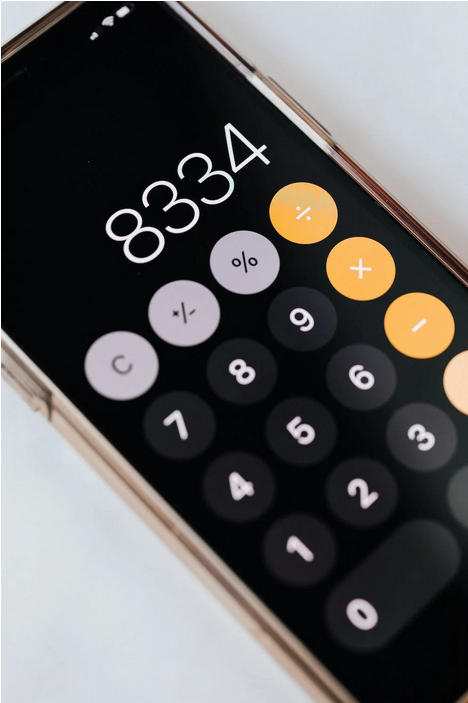
This code prints the sum of all numbers in the range of 0-9. The code uses a generator expression to iterate over the range and add each of the numbers in the range to the sum.
print(sum(i for i in range(10)))
Output:
45
One-Liner 6: Checking If Any Number in Range is Less Than Five
This code prints out a boolean value. It checks if any of the numbers in the range 0-9 are less than 5. The range starts at 0 and goes up to 9, so the answer is True
.
print(any(i < 5 for i in range(10)))
Output:
True
One-Liner 7: Checking if All Numbers in Range 0-9 are Even
This code prints True
if all elements in the given range of 0 to 10 are even (dividable by 2 with no remainder). The all()
function returns a boolean value, in this case it evaluates the expression “i % 2 == 0
” for each element in the given range (0 to 10) and returns True
if the expression is True
for all elements in the range.
print(all(i % 2 == 0 for i in range(10)))
Output:
False
One-Liner 8: Find Minimum Value of Range 0-9 with One Line of Code
This code prints the minimum value in the range 0 to 9 (inclusive). The output of this code would be 0. The code uses a generator expression to iterate over the range 0 to 9, and the min()
function is used to get the minimum value from the generated list.
print(min(i for i in range(10)))
Output:
0
One-Liner 9: Find Maximum Value in Range of 0 to 9
This code prints the maximum number in a range of ten numbers (0-9). The max()
function takes an argument of an iterable, in this case a generator expression (i for i in range(10)
). This generator expression creates a sequence of numbers from 0 to 9, which is then passed to the max()
function, which returns the maximum number in the sequence (9
).
print(max(i for i in range(10)))
Output:
9
One-Liner 10: Sort Numbers from 0 to 9 in Ascending Order
This code creates a list of numbers from 0 to 9 and sorts them in ascending order using the sorted()
built-in function.
print(sorted(i for i in range(10)))
Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
One-Liner 11: Print All Even Numbers from 0 to 10
This code prints a list of all even numbers from 0 to 9. The list comprehension syntax is used to create the list. The range(10)
function creates a sequence from 0 to 9. The if
statement checks to see if the number is even (i % 2 == 0
) and if it is, it is added to the list.
print([i for i in range(10) if i % 2 == 0])
Output:
[0, 2, 4, 6, 8]
One-Liner 12: Generate Dictionary of Squared Even Numbers Up to 10

This code prints a dictionary containing keys 0-9 and values 0-81. The keys and values are generated by looping through the range 0-9 and squaring each number if it is even (i.e., if the remainder of the number divided by 2 is 0). This is known as a dictionary comprehension. The result is a dictionary where the keys are even numbers 0-9 and the values are the squares of the even numbers 0-9.
print({i: i**2 for i in range(10) if i % 2 == 0})
Output:
{0: 0, 2: 4, 4: 16, 6: 36, 8: 64}
One-Liner 13: Sum of All Even Numbers from 0 to 9
This code calculates the sum of all even numbers from 0 to 9 (inclusive). The range()
function creates a sequence of numbers from 0 to 9. The if
statement checks if the number is even (i % 2 == 0
) and if so, adds it to the sum.
print(sum(i for i in range(10) if i % 2 == 0))
Output:
20
One-Liner 14: Even Numbers Less Than 5 Found in Range 0-9
This code prints “True
” to the console. It uses the any()
function to check if any item in the range of 0 to 9 that is divisible by two is less than 5. Since the result is true (2, 4, and 0 are less than 5), the function returns True
.
print(any(i < 5 for i in range(10) if i % 2 == 0))
Output:
True
One-Liner 15: Verifying if All Even Numbers in Range of 0 to 10 are Multiples of 3
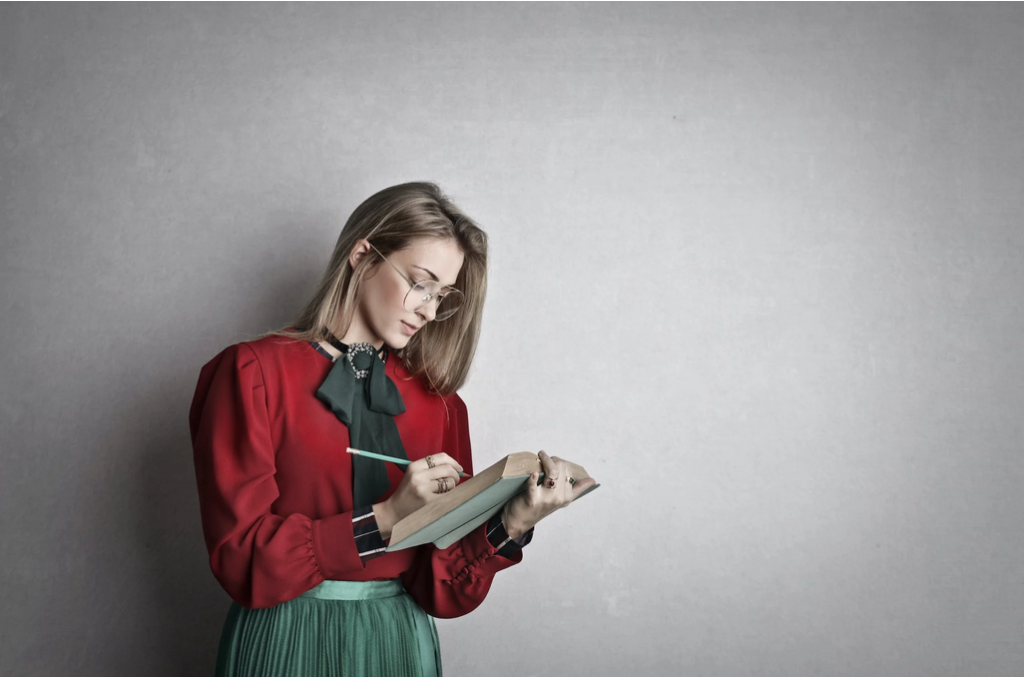
This code uses the all()
function which takes an iterable
as an argument and returns True
if all elements of the iterable are true (or if the iterable is empty). In this case, the iterable is a generator expression which uses a for
loop to iterate over the numbers 0-9 and check if they are divisible by 3. The if
statement filters out the odd numbers, so the all()
function only checks if the remaining even numbers are divisible by 3. Since they all are, the code prints True
.
print(all(i % 3 == 0 for i in range(10) if i % 2 == 0))
Output:
False
One-Liner 16: Find Minimum Even Number in Range 0-9
In this code, the min()
function is used to return the minimum value in the given sequence. The sequence is a generator expression, which creates a generator object with values that satisfy the given condition (i % 2 == 0
). This means that the sequence will contain all numbers between 0 and 9 that are divisible by 2. The min()
function will then return the smallest number in this sequence, which is 0.
print(min(i for i in range(10) if i % 2 == 0))
Output:
0
One-Liner 17: Calculate Maximum Even Number in Range 0-9
This code prints the maximum even number from 0-10. The code uses a generator expression to check for even numbers (i % 2 == 0
) in the range 0-10, and the max()
function to find the maximum value. The output of this code is 8.
print(max(i for i in range(10) if i % 2 == 0))
Output:
8
One-Liner 18: Print Even Numbers from 0-9 in Ascending Order
This code prints a list of numbers from 0 to 9, but only the even numbers. The list is sorted in ascending order. The code uses a generator expression to create a list of numbers from 0 to 9. The “if
” statement filters out the odd numbers from the list and the sorted()
function sorts the remaining even numbers in ascending order.
print(sorted(i for i in range(10) if i % 2 == 0))
Output:
[0, 2, 4, 6, 8]
One-Liner 19: Printing Squares of the First Ten Natural Numbers
This code uses a for
loop and f-strings to print the squares of the numbers from 0 to 9. For each number in the range 0 to 9, the code prints a string which includes the number, followed by the multiplication symbol *
, followed by the number again, followed by an equals sign, followed by the square of the number.
[print(f"{i} * {i} = {i*i}") for i in range(10)]
Output:
0 * 0 = 0
1 * 1 = 1
2 * 2 = 4
3 * 3 = 9
4 * 4 = 16
5 * 5 = 25
6 * 6 = 36
7 * 7 = 49
8 * 8 = 64
9 * 9 = 81
One-Liner 20: Printing a Dictionary Comprehension of Squares of Numbers 0-9
This code prints a dictionary with keys and values in the form of squared integers ranging from 0 to 9. The dictionary comprehension syntax consists of an expression pair (key: value
) followed by a for
statement inside curly brackets {}
. The expression pair is the key i
and the value i**2
(i
squared). The for
statement iterates through the range of integers from 0 to 9.
print({i: i**2 for i in range(10)})
The code prints a dictionary that looks like this:
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
One-Liner 21: Printing Integers 0-9 on Separate Lines
This code will print out the numbers 0 through 9 on separate lines. The code uses a for
loop to iterate through the range of 0-9 and print out the value of the variable i
for each iteration.
for i in range(10): print(i)
Output:
0
1
2
3
4
5
6
7
8
9
One-Liner 22: Printing Numbers 0-9 in Python
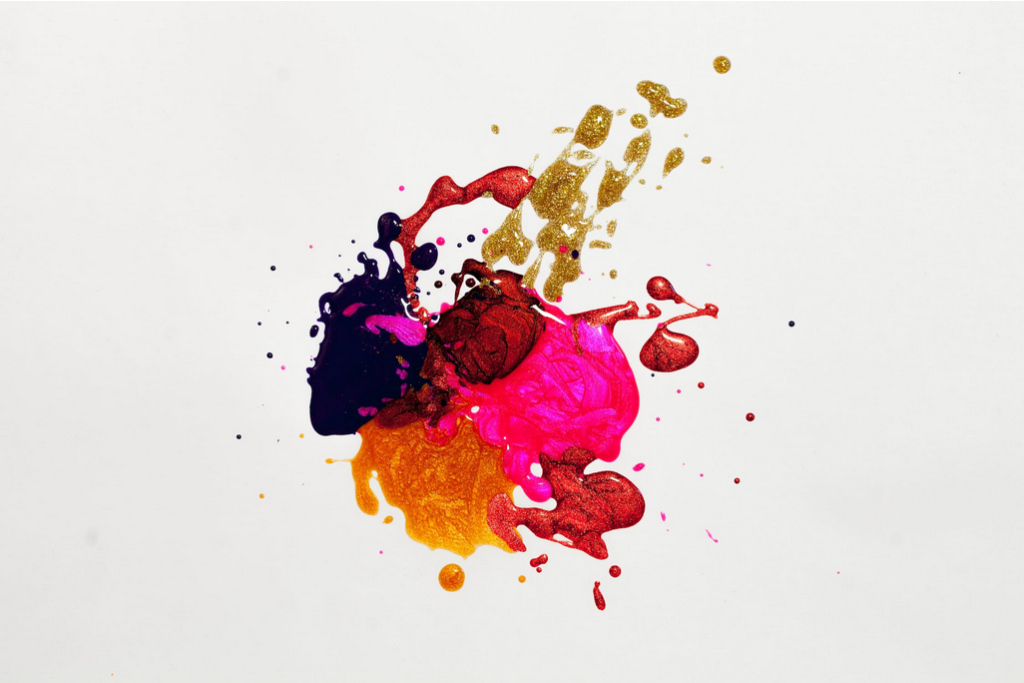
This code is a for
loop that prints the numbers 0-9 on a single line. The code uses a list comprehension, which is a succinct way to create a list. In this example, the code creates a list of numbers 0-9 and then prints each number on a single line with a space between them. The end=" "
parameter tells the program to add a space after each number instead of a new line.
[print(i, end=" ") for i in range(10)]
Output:
0 1 2 3 4 5 6 7 8 9]
One-Liner 23: Printing the Range from 0 to 9
This code prints out the numbers 0-9. The asterisk (*
) is used to unpack the range()
function so that the numbers in the range can be printed out one at a time.
print(*range(10))
Output:
0 1 2 3 4 5 6 7 8 9
One-Liner 24: Printing Values of Squared Integers from 0 to 9
This code is using a dictionary comprehension to create a dictionary with the keys being the numbers 0-9 and the values being the squares of the numbers 0-9. It then prints the values of the dictionary. The output would be a dictionary view object containing the values 0, 1, 4, 9, 16, 25, 36, 49, 64, 81.
print({i: i**2 for i in range(10)}.values())
Output:
dict_values([0, 1, 4, 9, 16, 25, 36, 49, 64, 81])
One-Liner 25: Generate a Dictionary of Squared Integers from 0 to 9 and Print the Keys
This code prints the keys of a dictionary where the keys are the numbers 0-9 and the values are the squares of the keys.
print({i: i**2 for i in range(10)}.keys())
Output:
dict_keys([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
One-Liner 26: Printing Key-Value Pairs of Squares from 0 to 9
This code prints out the items in a dictionary as a list of tuples. The dictionary is created using a dictionary comprehension, which creates a dictionary with keys ranging from 0 to 9 (i
) and values corresponding to the square of each key (i**2
). The print()
statement uses the asterisk operator to unpack the items of the dictionary, which are then printed out as a list of tuples.
print(*{i: i**2 for i in range(10)}.items())
Output:
(0, 0) (1, 1) (2, 4) (3, 9) (4, 16) (5, 25) (6, 36) (7, 49) (8, 64) (9, 81)
One-Liner 27: Printing the Squares of Numbers 0-9
This code prints the squares of all numbers from 0 to 9. It uses a list comprehension to first generate a list of squares of all numbers from 0 to 9. Then, it uses a for
loop to loop through this list and prints each item on the list, with a space between each item.
[print(i, end=" ") for i in [i**2 for i in range(10)]]
Output:
0 1 4 9 16 25 36 49 64 81
One-Liner 28: Print Powers of Ten with a Loop

This code will print the squares of numbers from 0 to 9. The for
loop will iterate through the range of 0 to 9, and for each iteration, the exec()
function will execute the command within its argument. The command within the exec()
function is a f-string that prints out a number raised to the power of two.
for i in range(10): exec(f"print({i} ** 2)")
Output:
0
1
4
9
16
25
36
49
64
81
One-Liner 29: Printing Squares of Numbers from 0 to 9
This code prints the first 10 square numbers (0-9) to the console. The print()
function is used to output the result of the generator expression, which uses the asterisk (*
) operator to unpack the sequence of values. The generator expression creates a sequence of values by looping through the range(10)
function and raising each number to the power of 2.
print(*(i**2 for i in range(10)))
Output:
0 1 4 9 16 25 36 49 64 81
One-Liner 30: Generate a List of Squares from 0 to 9
This code prints a list containing the squares of the numbers 0-9. The code uses a generator expression to create a generator object, which contains the squares of the numbers 0-9. This generator object is then converted to a list and printed.
print(list(i**2 for i in range(10)))
Output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
One-Liner 31: Printing a Dictionary with the Squares of Integers from 0 to 9
This code prints a dictionary of 10 key-value pairs. The keys are numbers from 0-9, and the values are the squares of those numbers. The zip()
function creates an iterator of the two arguments, which in this case are range(10)
and a generator expression (i**2 for i in range(10)
) that produces the squares of the numbers in the range. The dict()
function then creates a dictionary from the resulting iterator.
print(dict(zip(range(10), (i**2 for i in range(10)))))
Output:
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
One-Liner 32: Printing the Square of the Fifth Integer in a Range of Ten

This code is using a dictionary comprehension to create a dictionary with key-value pairs where the key is a number from 0 to 9 and the value is the square of the key. It then prints the value of the key 5, which is 25.
print({i: i**2 for i in range(10)}[5])
Output:
25
One-Liner 33: Printing a List of Tuples of Range and Squared Values
This code prints a list of tuples, where each tuple contains a number from 0 to 9 and the square of that number. The range()
function is used to create a sequence of numbers from 0 to 9, and the list comprehension (i**2 for i in range(10)
) is used to create a sequence of the squares of those numbers. The zip()
function combines the two sequences into a list of tuples.
print(list(zip(range(10), (i**2 for i in range(10)))))
Output:
[(0, 0), (1, 1), (2, 4), (3, 9), (4, 16), (5, 25), (6, 36), (7, 49), (8, 64), (9, 81)]
One-Liner 34: Print a List of Cubed Numbers from 0 to 9
This code uses the map()
function and a lambda expression to create a list of the cubes of all numbers from 0 to 9. The map()
function applies the given lambda expression to each item in the range, which is 0 to 9 in this case. The lambda expression takes the value of the item, x
, and cubes it, x**3
. Finally, the list()
function is used to convert the map object returned by map()
into a list. The output of this code is a list containing the cubes of 0-9.
print(list(map(lambda x: x**3, range(10))))
Output:
[0, 1, 8, 27, 64, 125, 216, 343, 512, 729]
One-Liner 35: Generate a List of Powers of 10 from 0 to 9
This code prints the resulting list of raising the numbers in the range 0-9 to the power of 2. The map()
function takes two arguments – the first is the function to apply, in this case pow()
, and the second is the iterable. The range(10)
argument creates an iterable object of numbers 0-9 and the [2]*10
creates a list of 10 2
s. Thus, the pow()
function is applied to each number in the range 0-9, raised to the power of the list’s corresponding number, which is 2.
print(list(map(pow, range(10), [2]*10)))
Output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
One-Liner 36: Generating and Manipulating a List of Squares from 0 to 9
This code creates a list of the squares of the numbers 0-9 and prints out the 6th element, the first 5 elements, the elements from the 6th element to the end of the list, the elements from the 4th element to the 7th element, and the elements from the start of the list to the end of the list, in steps of 2.
l = [i**2 for i in range(10)] print(l[5]) print(l[:5]) print(l[5:]) print(l[3:7]) print(l[::2])
Output:
25
[0, 1, 4, 9, 16]
[25, 36, 49, 64, 81]
[9, 16, 25, 36]
[0, 4, 16, 36, 64]
One-Liner 37: Creating and Accessing Lists of Squares of Numbers from 0 to 9

This code creates a list of lists containing the numbers from 0 to 9 and their squares. It then prints the 6th element of the list (the sixth number and its square), the first five elements of the list, the elements from the 6th element to the end of the list, the elements from the 4th element to the 7th element, and then the elements from the list in steps of two.
l2 = [[i, i**2] for i in range(10)] print(l2[5]) print(*l2[:5]) print(*l2[5:]) print(*l2[3:7]) print(*l2[::2])
Output:
[5, 25]
[0, 0] [1, 1] [2, 4] [3, 9] [4, 16]
[5, 25] [6, 36] [7, 49] [8, 64] [9, 81]
[3, 9] [4, 16] [5, 25] [6, 36]
[0, 0] [2, 4] [4, 16] [6, 36] [8, 64]
One-Liner 38: Generating a Dictionary of Squared Values from 0 to 9
This code is creating a dictionary that stores the value of each number from 0 to 9 squared. The result will be a dictionary of key-value pairs, where the key is a number from 0 to 9 and the value is that number squared.
print({i: i**2 for i in range(10)})
Output:
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills. You’ll learn about advanced Python features such as list comprehension, slicing, lambda functions, regular expressions, map and reduce functions, and slice assignments.
You’ll also learn how to:
- Leverage data structures to solve real-world problems, like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array, shape, axis, type, broadcasting, advanced indexing, slicing, sorting, searching, aggregating, and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups, negative lookaheads, escaped characters, whitespaces, character sets (and negative characters sets), and greedy/nongreedy operators
- Understand a wide range of computer science topics, including anagrams, palindromes, supersets, permutations, factorials, prime numbers, Fibonacci numbers, obfuscation, searching, and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined, and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
December 13, 2022 at 03:39PM
Click here for more details...
=============================
The original post is available in Finxter by Sensei
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
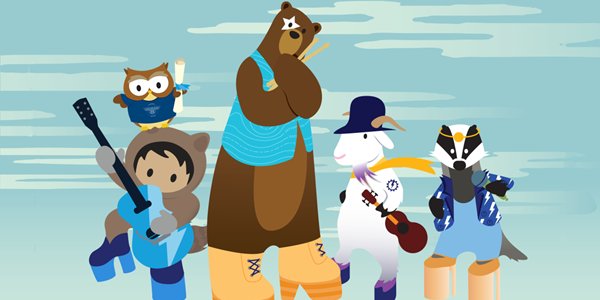
Post a Comment