Python | Split String by Number : Shubham Sayon
by: Shubham Sayon
blow post content copied from Finxter
click here to view original post
Summary: To split a string by a number, use the regex
split
method using the “\d
” pattern.
Minimal Example
my_string = "#@1abc3$!*5xyz" # Method 1 import re res = re.split('\d+', my_string) print(res) # Method 2 import re res = re.findall('\D+', my_string) print(res) # Method 3 from itertools import groupby li = [''.join(g) for _, g in groupby(my_string, str.isdigit)] res = [x for x in li if x.isdigit() == False] print(res) # Method 4 res = [] for i in my_string: if i.isdigit() == True: my_string = my_string.replace(i, ",") print(my_string.split(",")) # Outputs: # ['#@', 'abc', '$!*', 'xyz']
Problem Formulation
Problem: Given a string containing different characters. How will you split the string whenever a number appears?
Method 1: re.split()
The re.split(pattern, string)
method matches all occurrences of the pattern
in the string
and divides the string along the matches resulting in a list of strings between the matches. For example, re.split('a', 'bbabbbab')
results in the list of strings ['bb', 'bbb', 'b']
.
Code:
import re my_string = "#@1abc3$!*5xyz" res = re.split('\d+', my_string) print(res) # ['#@', 'abc', '$!*', 'xyz']
Explanation: The \d
special character matches any digit between 0 and 9. By using the maximal number of digits as a delimiter, you split along the digit-word boundary.
Method 2: re.findall()
The re.findall(pattern, string)
method scans string
from left to right, searching for all non-overlapping matches of the pattern
. It returns a list of strings in the matching order when scanning the string from left to right.
Code:
import re my_string = "#@1abc3$!*5xyz" res = re.findall('\D+', my_string) print(res) # ['#@', 'abc', '$!*', 'xyz']
Explanation: The \
D special character matches all characters except any digit between 0 and 9. Thus, you are essentially finding all character groups that appear before the occurrence of a digit.
Do you want to master the regex superpower? Check out my new book The Smartest Way to Learn Regular Expressions in Python with the innovative 3-step approach for active learning: (1) study a book chapter, (2) solve a code puzzle, and (3) watch an educational chapter video.
Method 3: itertools.groupby()
Code:
from itertools import groupby my_string = "#@1abc3$!*5xyz" li = [''.join(g) for _, g in groupby(my_string, str.isdigit)] res = [x for x in li if x.isdigit() == False] print(res) # ['#@', 'abc', '$!*', 'xyz']
Explanation:
- The
itertools.groupby(iterable, key=None)
function creates an iterator that returns tuples(key, group-iterator)
grouped by each value ofkey
. We use thestr.isdigit()
function as key function. - The
str.isdigit()
function returnsTrue
if the string consists only of numeric characters. Thus, you will have a list created by using numbers as separators. Note that this list will also contain the numbers as items within it. - In order to eliminate the numbers, use another list comprehension that checks if an element in the list returned previously is a digit or not with the help of the
isdigit
method. If it is a digit, the item will be discarded. Otherwise it will be stored in the list.
Method 4: Replace Using a for Loop
Approach: Use a for loop to iterate through the characters of the given string. Check if a character is a digit or not. As soon as a digit is found, replace that character/digit with a delimiter string ( we have used a comma here) with the help of the replace() method. This basically means that you are placing a particular character in the string whenever a number appears. Once all the digits are replaced by the separator string, split the string by passing the separator string as a delimiter to the split method.
Code:
my_string = "#@1abc3$!*5xyz" res = [] for i in my_string: if i.isdigit(): my_string = my_string.replace(i, ",") print(my_string.split(",")) # ['#@', 'abc', '$!*', 'xyz']
Conclusion
Phew! We have successfully solved the given problem and managed to do so using four different ways. I hope you found this article helpful and it answered your queries. Please subscribe and stay tuned for more solutions and tutorials.
Happy coding!
Related Read: How to Split a String Between Numbers and Letters?
December 03, 2022 at 11:35PM
Click here for more details...
=============================
The original post is available in Finxter by Shubham Sayon
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
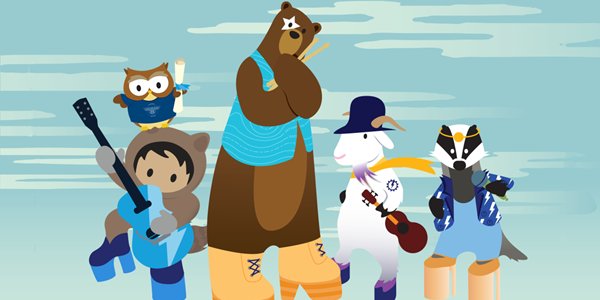
Post a Comment