John Ludhi/nbshare.io: Generative Adversarial Networks :
by:
blow post content copied from Planet Python
click here to view original post
Generative Adversarial Networks (GANs) are a type of neural network architecture for generative modeling. They consist of two models: a generator and a discriminator. The generator produces synthetic data samples that are intended to be indistinguishable from real data, while the discriminator is trained to distinguish between real and synthetic data samples.
The generator and discriminator are trained together in an adversarial process. The generator tries to produce synthetic data that the discriminator will classify as real, while the discriminator tries to correctly classify real and synthetic data. This process continues until the generator produces synthetic data that is realistic enough to fool the discriminator.
One of the key benefits of GANs is that they can learn to generate synthetic data that is highly realistic and diverse. This has made them particularly popular for tasks such as image generation, text generation, and audio synthesis.
An example of synthetic data generated by a GAN might be a set of realistic images of objects or scenes that do not actually exist in the real world. For example, a GAN might be trained on a large dataset of real images of faces and then be able to generate synthetic images of new, previously unseen faces that are highly realistic and diverse.
Real data, on the other hand, is data that is collected from the real world and is not artificially generated. For example, a dataset of real images of faces might be collected by taking photographs of people and using those images as the training data for a machine learning model.
Generative models are a type of machine learning model that is capable of generating new data samples that are similar to a training dataset. The goal of a generative model is to learn the underlying distribution of the training data and use this knowledge to generate new, synthetic data samples.
GANs are a specific type of generative model that uses a two-part architecture consisting of a generator and a discriminator as explained above. There are other types of generative models besides GANs, such as Variational Autoencoders (VAEs) and Normalizing Flow models. These models use different techniques to learn the underlying distribution of the training data and generate synthetic data samples.
In a Generative Adversarial Network (GAN), the generator is a neural network that is trained to generate synthetic data samples that are intended to be indistinguishable from real data. The generator is typically trained by being given random noise as input and using this noise to generate a synthetic data sample.
The specific architecture and design of the generator model can vary depending on the type of data it is generating. For example, a GAN trained to generate images might use a convolutional neural network (CNN) as the generator, while a GAN trained to generate text might use a recurrent neural network (RNN) as the generator.
import warnings
warnings.filterwarnings('ignore')
import numpy as np
import keras
from keras import layers
latent_dim = 32
height = 32
width = 32
channels = 3
generator_input = keras.Input(shape=(latent_dim,))
x = layers.Dense(128 * 16 * 16)(generator_input)
x = layers.LeakyReLU()(x)
x = layers.Reshape((16, 16, 128))(x)
x = layers.Conv2D(256, 5, padding='same')(x)
x = layers.LeakyReLU()(x)
x = layers.Conv2DTranspose(256, 4, strides=2, padding='same')(x)
x = layers.LeakyReLU()(x)
x = layers.Conv2D(256, 5, padding='same')(x)
x = layers.LeakyReLU()(x)
x = layers.Conv2D(256, 5, padding='same')(x)
x = layers.LeakyReLU()(x)
x = layers.Conv2D(channels, 7, activation='tanh', padding='same')(x)
generator = keras.models.Model(generator_input, x)
generator.summary()
Model: "model_1" _________________________________________________________________ Layer (type) Output Shape Param # ================================================================= input_2 (InputLayer) [(None, 32)] 0 dense_1 (Dense) (None, 32768) 1081344 leaky_re_lu_5 (LeakyReLU) (None, 32768) 0 reshape_1 (Reshape) (None, 16, 16, 128) 0 conv2d_4 (Conv2D) (None, 16, 16, 256) 819456 leaky_re_lu_6 (LeakyReLU) (None, 16, 16, 256) 0 conv2d_transpose_1 (Conv2DT (None, 32, 32, 256) 1048832 ranspose) leaky_re_lu_7 (LeakyReLU) (None, 32, 32, 256) 0 conv2d_5 (Conv2D) (None, 32, 32, 256) 1638656 leaky_re_lu_8 (LeakyReLU) (None, 32, 32, 256) 0 conv2d_6 (Conv2D) (None, 32, 32, 256) 1638656 leaky_re_lu_9 (LeakyReLU) (None, 32, 32, 256) 0 conv2d_7 (Conv2D) (None, 32, 32, 3) 37635 ================================================================= Total params: 6,264,579 Trainable params: 6,264,579 Non-trainable params: 0 _________________________________________________________________
As you can see in the above code, There are multiple LeakyReLU and dense layers in the GAN generator model in the above code because they allow the model to learn a hierarchy of features from the input noise.
To learn more about Activation functions, checkout following links...
https://www.nbshare.io/notebook/751082217/Activation-Functions-In-Python/
https://www.nbshare.io/notebook/626290365/What-is-LeakyReLU-Activation-Function/
In a deep learning model, the layers closer to the input are responsible for learning lower-level features (e.g., edges, corners), while the layers closer to the output are responsible for learning higher-level features (e.g., shapes, objects). By stacking multiple layers, the model can learn a hierarchy of features at different levels of abstraction, which can help it generate more realistic images.
The dense layers in the generator model learn to transform the input noise into a high-dimensional feature space, which is then upsampled by the convolutional layers to generate the output image. The LeakyReLU activation function is used in between the layers to introduce non-linearity into the model, which can help it learn more complex relationships between the input and output.
The specific number and arrangement of layers in the generator model can vary depending on the complexity of the task and the size of the input and output data. Experimenting with different architectures can sometimes lead to better model performance.
The discriminator model in a GAN is a neural network that takes an image as input and outputs a probability that the image is real (as opposed to synthetic). The discriminator model is trained to maximize the probability of correctly classifying real images as real, and synthetic images as synthetic.
import numpy as np
import keras
from keras import layers
height = 32
width = 32
channels = 3
discriminator_input = keras.Input(shape=(height, width, channels))
x = layers.Conv2D(128, 3)(discriminator_input)
x = layers.LeakyReLU()(x)
x = layers.Conv2D(128, 4, strides=2)(x)
x = layers.LeakyReLU()(x)
x = layers.Conv2D(128, 4, strides=2)(x)
x = layers.LeakyReLU()(x)
x = layers.Conv2D(128, 4, strides=2)(x)
x = layers.LeakyReLU()(x)
x = layers.Flatten()(x)
x = layers.Dropout(0.4)(x)
x = layers.Dense(1, activation='sigmoid')(x)
discriminator = keras.models.Model(discriminator_input, x)
discriminator.summary()
# To stabilize training, we use learning rate decay
# and gradient clipping (by value) in the optimizer.
discriminator_optimizer = keras.optimizers.RMSprop(lr=0.0008, clipvalue=1.0, decay=1e-8)
discriminator.compile(optimizer=discriminator_optimizer, loss='binary_crossentropy')
Model: "model_2" _________________________________________________________________ Layer (type) Output Shape Param # ================================================================= input_3 (InputLayer) [(None, 32, 32, 3)] 0 conv2d_8 (Conv2D) (None, 30, 30, 128) 3584 leaky_re_lu_10 (LeakyReLU) (None, 30, 30, 128) 0 conv2d_9 (Conv2D) (None, 14, 14, 128) 262272 leaky_re_lu_11 (LeakyReLU) (None, 14, 14, 128) 0 conv2d_10 (Conv2D) (None, 6, 6, 128) 262272 leaky_re_lu_12 (LeakyReLU) (None, 6, 6, 128) 0 conv2d_11 (Conv2D) (None, 2, 2, 128) 262272 leaky_re_lu_13 (LeakyReLU) (None, 2, 2, 128) 0 flatten (Flatten) (None, 512) 0 dropout (Dropout) (None, 512) 0 dense_2 (Dense) (None, 1) 513 ================================================================= Total params: 790,913 Trainable params: 790,913 Non-trainable params: 0 _________________________________________________________________
This discriminator model takes an image of shape (height, width, channels) as input and outputs a probability that the image is real.
The model consists of a series of convolutional layers that learn to extract features from the input image, followed by a dense layer that uses these features to classify the image as real or synthetic.
The discriminator model is trained using the binary cross-entropy loss function, which measures the distance between the predicted probability and the true label (real or synthetic). The model is optimized using the RMSprop optimizer, with learning rate decay and gradient clipping to stabilize training.
Note:
The sigmoid function is used as the activation function of the output layer of the discriminator model in the above code because it maps the output of the layer to a value between 0 and 1, which can be interpreted as a probability.
The binary cross-entropy loss function can then be used to measure the distance between the predicted probability and the true label (real or synthetic), and the model can be trained to minimize this distance.
January 08, 2023 at 07:08AM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
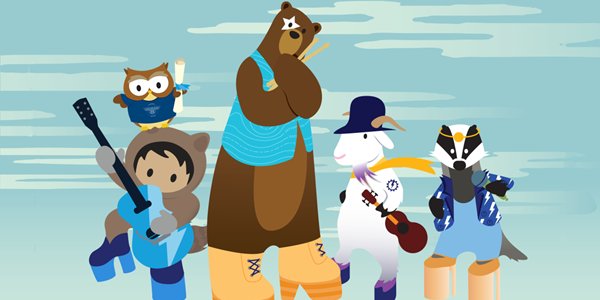
Post a Comment