Basic Data Types in Python: A Quick Exploration :
by:
blow post content copied from Real Python
click here to view original post
Python has several basic data types that are built into the language. With these types, you can represent numeric values, text and binary data, and Boolean values in your code. So, these data types are the basic building blocks of most Python programs and projects.
In this tutorial, you’ll learn about:
- Numeric types, such as
int
,float
, andcomplex
- The
str
data type, which represents textual data - The
bytes
andbytearray
data types for storing bytes - Boolean values with the
bool
data type
In this tutorial, you’ll learn only the basics of each data type. To learn more about a specific data type, you’ll find useful resources in the corresponding section.
Get Your Code: Click here to download the free sample code that you’ll use to learn about basic data types in Python.
Take the Quiz: Test your knowledge with our interactive “Basic Data Types in Python: A Quick Exploration” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Basic Data Types in Python: A Quick ExplorationTake this quiz to test your understanding of the basic data types that are built into Python, like numbers, strings, and Booleans.
Python’s Basic Data Types
Python has several built-in data types that you can use out of the box because they’re built into the language. From all the built-in types available, you’ll find that a few of them represent basic objects, such as numbers, strings and characters, bytes, and Boolean values.
Note that the term basic refers to objects that can represent data you typically find in real life, such as numbers and text. It doesn’t include composite data types, such as lists, tuples, dictionaries, and others.
In Python, the built-in data types that you can consider basic are the following:
Class | Basic Type |
---|---|
int |
Integer numbers |
float |
Floating-point numbers |
complex |
Complex numbers |
str |
Strings and characters |
bytes , bytearray |
Bytes |
bool |
Boolean values |
In the following sections, you’ll learn the basics of how to create, use, and work with all of these built-in data types in Python.
Integer Numbers
Integer numbers are whole numbers with no decimal places. They can be positive or negative numbers. For example, 0
, 1
, 2
, 3
, -1
, -2
, and -3
are all integers. Usually, you’ll use positive integer numbers to count things.
In Python, the integer data type is represented by the int
class:
>>> type(42)
<class 'int'>
In the following sections, you’ll learn the basics of how to create and work with integer numbers in Python.
Integer Literals
When you need to use integer numbers in your code, you’ll often use integer literals directly. Literals are constant values of built-in types spelled out literally, such as integers. Python provides a few different ways to create integer literals. The most common way is to use base-ten literals that look the same as integers look in math:
>>> 42
42
>>> -84
-84
>>> 0
0
Here, you have three integer numbers: a positive one, a negative one, and zero. Note that to create negative integers, you need to prepend the minus sign (-
) to the number.
Python has no limit to how long an integer value can be. The only constraint is the amount of memory your system has. Beyond that, an integer can be as long as you need:
>>> 123123123123123123123123123123123123123123123123 + 1
123123123123123123123123123123123123123123123124
For a really, really long integer, you can get a ValueError
when converting it to a string:
>>> 123 ** 10000
Traceback (most recent call last):
...
ValueError: Exceeds the limit (4300 digits) for integer string conversion;
use sys.set_int_max_str_digits() to increase the limit
Read the full article at https://realpython.com/python-data-types/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
May 20, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
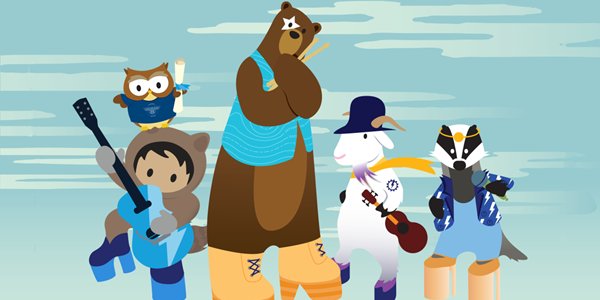
Post a Comment