Python Beginner Cheat Sheet: 19 Keywords Every Coder Must Know : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Feeling lost in the sea of Python syntax and libraries? You’re not alone!
More than 200,000 absolute Python beginners have already downloaded our free cheat sheets — and grown to a coding level that empowered them to build cool apps!
Why did I create the cheat sheets? Initially for myself. As more and more people checked them out, I started formulating an explicit goal:
My Goal: Minimize your time and effort needed to go from zero Python skills to creating your first cool app.
Can we get the basics done in 10 minutes? You be the judge! Let’s go!
Python Keywords Cheat Sheet
This cheat sheet is for beginners in the Python programming language. It explains everything you need to know about Python keywords.
Download and pin it to your wall until you feel confident using all these keywords!
Over time, this page has turned into a full-fledged Python tutorial with many additional resources, puzzles, tips, and videos. Go ahead—have some fun & try to learn a thing or two & become a better coder in the process!
Interactive Python Puzzle
I’ve written a short puzzle that incorporates all keywords discussed in the cheat sheet. Can you solve it?
Interactive Puzzle Link: https://app.finxter.com/learn/computer/science/653
Exercise: Think about this puzzle and guess the output. Then, check whether you were right!
Did you struggle with the puzzle? No problem — Let’s dive into all of these keywords to gain a better understanding of each.
Python Keywords
Learn 80% of the keywords in 20% of the time: these are the most important Python keywords.
False, True
Data values from the Boolean data type
False == (1 > 2) True == (2 > 1)
and, or, not
Logical operators:
(x and y)
→ bothx
andy
must beTrue
for the expression to beTrue
(x or y)
→ eitherx
ory
or both must beTrue
for the expression to beTrue
(not x)
→x
must beFalse
for the expression to beTrue
x, y = True, False (x or y) == True # True (x and y) == False # True (not y) == True # True
break
Ends loop prematurely
while(True): break # no infinite loop print("hello world")
continue
Finishes current loop iteration
while(True): continue print("43") # dead code
class
Defines a new class → a real-world concept
class Beer: def __init__(self): self.content = 1.0 def drink(self): self.content = 0.0 becks = Beer() # constructor - create class becks.drink() # beer empty: b.content == 0
def
Defines a new function or class method. For the latter, the first parameter (“self
”) points to the class object. When calling the class method, the first parameter is implicit.
See previous code example.
if, elif, else
Conditional program execution: program starts with “if
” branch, tries the “elif
” branches, and finishes with “else
” branch (until one branch evaluates to True
).
x = int(input("your value: ")) if x > 3: print("Big") elif x == 3: print("Medium") else: print("Small")
for, while
Repeated execution of loop body.
# For loop declaration for i in [0,1,2]: print(i) # While loop - same semantics j = 0 while j < 3: print(j) j = j + 1
in
Checks whether element is in sequence (membership):
42 in [2, 39, 42] # True
is
Checks whether both elements point to the same object (object identity/equality)
y = x = 3 x is y # True [3] is [3] # False
None
Empty value constant
def f(): x = 2 f() is None # True
lambda
Function with no name (anonymous function)
(lambda x: x + 3)(3) # returns 6
return
Terminates execution of the function and passes the flow of execution to the caller. An optional value after the return keyword specifies the function result.
def incrementor(x): return x + 1 incrementor(4) # returns 5
Put yourself on the road to mastery and download your free Python cheat sheets now, print them, and post them to your office wall!
Want more cheat sheets and free Python education? Register for the free Finxter email academy.
Go to the Next Level: If you really want to advance your career, even if you’re an absolute beginner, check out the Finxter Academy! You’ll learn the most important skill of our decade: using AI to create value. Each of our courses comes with a course certificate to get your dream job!
In the following, I’ll present you a compilation of the best Python cheat sheets on the web. So, keep reading!
Best Python Cheat Sheets
But these are not all—the following Python cheat sheets will greatly improve your learning efficiency! Check out this compilation of the best Python cheat sheets!
So let’s dive into the best Python cheat sheets recommended by us.
Python 3 Cheat Sheet
This is the best single cheat sheet. It uses every inch of the page to deliver value and covers everything you need to know to go from beginner to intermediate. Topics covered include container types, conversions, modules, math, conditionals, and formatting to name a few. A highly recommended 2-page sheet!
Python Beginner Cheat Sheet
Some might think this cheat sheet is a bit lengthy. At 26 pages, it is the most comprehensive cheat sheets out there. It explains variables, data structures, exceptions, and classes – to name just a few. If you want the most thorough cheat sheet, pick this one.
Python for Data Science
Some of the most popular things to do with Python are Data Science and Machine Learning.
In this cheat sheet, you will learn the basics of Python and the most important scientific library: NumPy (Numerical Python). You’ll learn the basics plus the most important NumPy functions.
If you are using Python for Data Science, download this cheat sheet.
Python for Data Science (Importing Data)
This Python data science cheat sheet from DataCamp is all about getting data into your code.
Think about it: importing data is one of the most important tasks when working with data. Increasing your skills in this area will make you a better data scientist—and a better coder overall!
Python Cheatography Cheat Sheet
This cheat sheet is for more advanced learners. It covers class, string, and list methods as well as system calls from the sys
module.
Once you’re comfortable defining basic classes and command-line interfaces (CLIs), get this cheat sheet. It will take you to another level.
The Ultimative Python Cheat Sheet Course (5x Email Series)
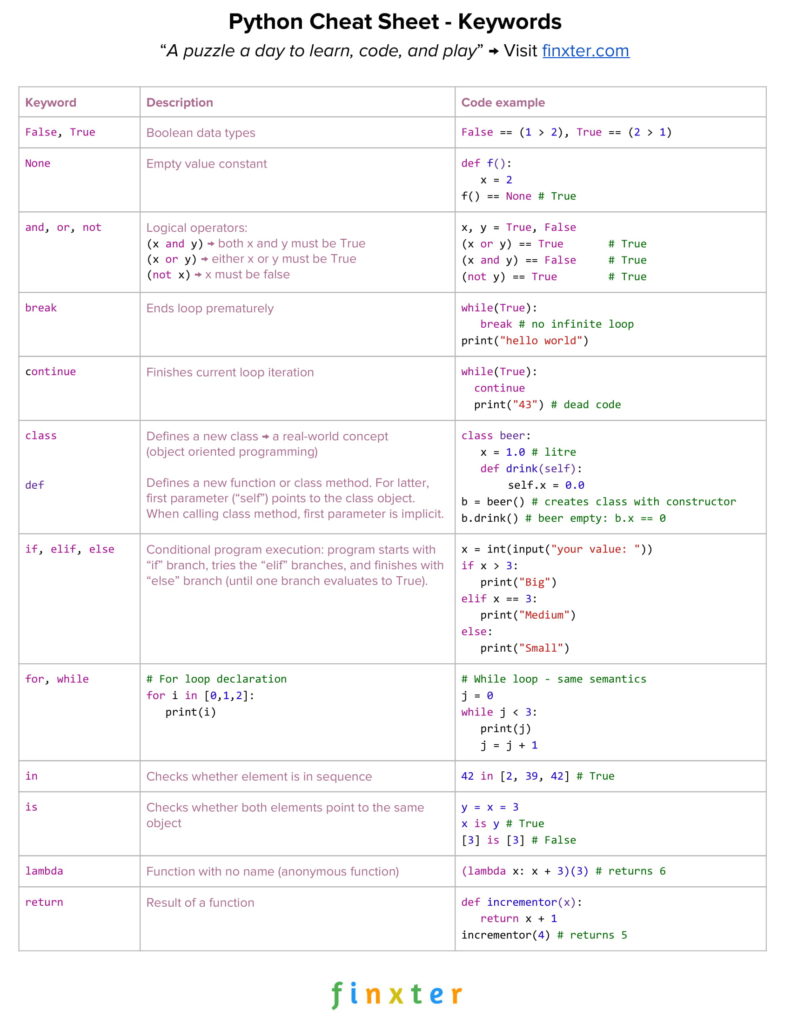

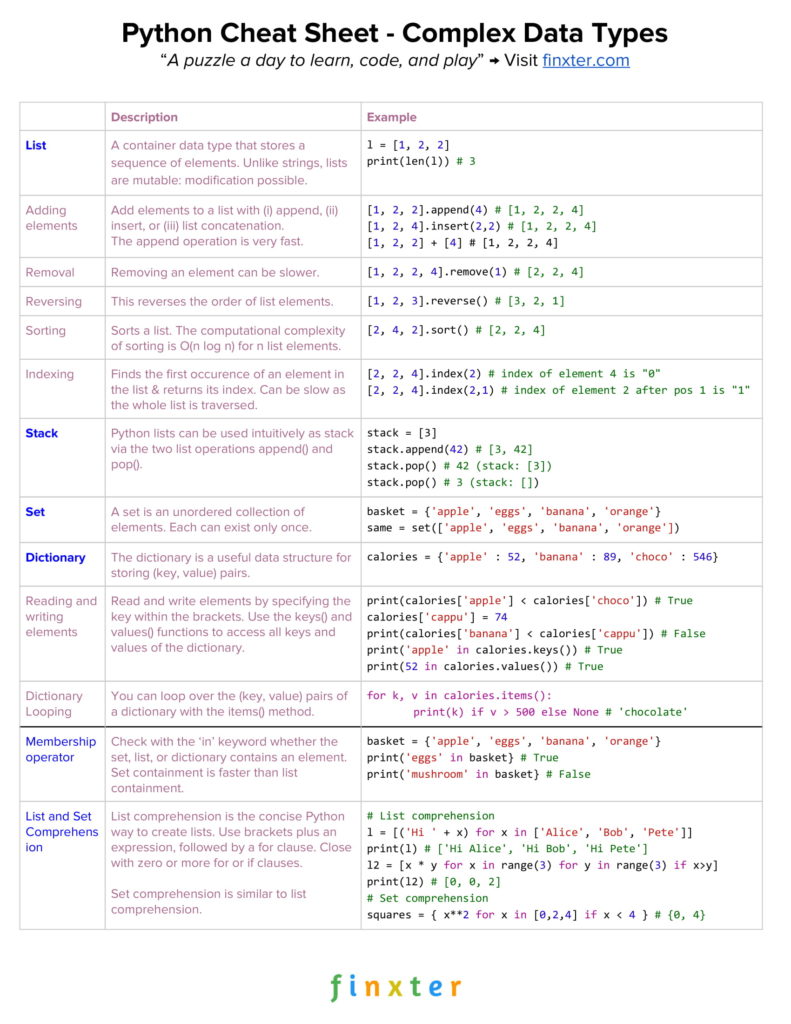
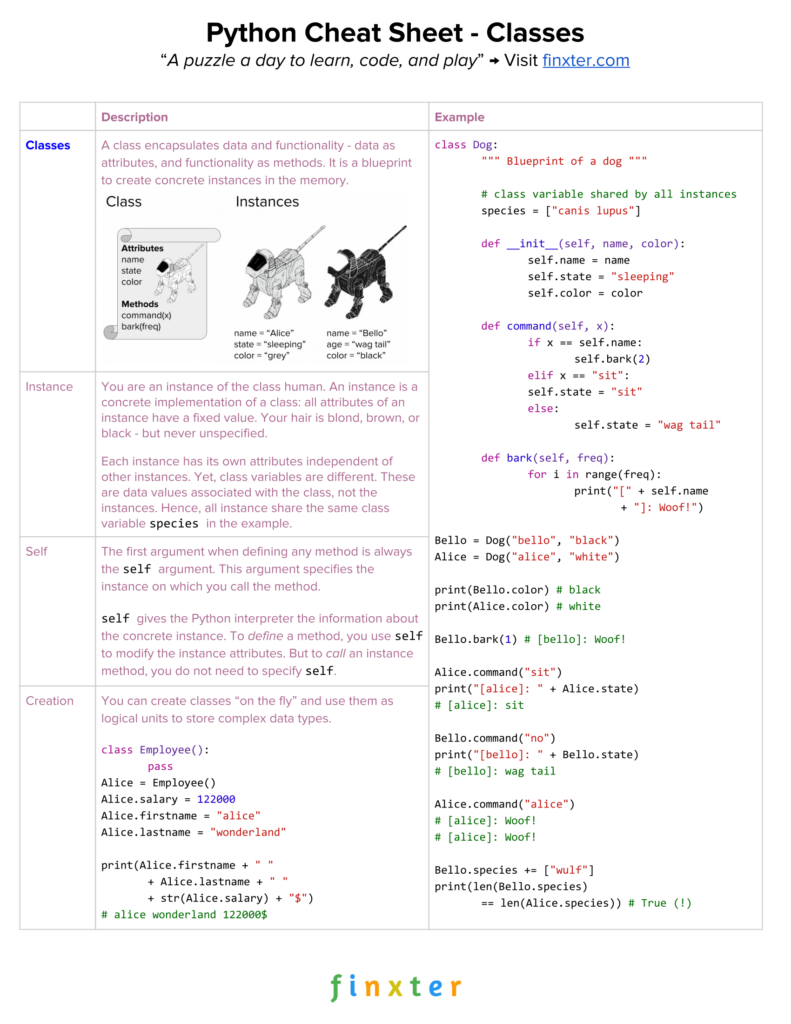
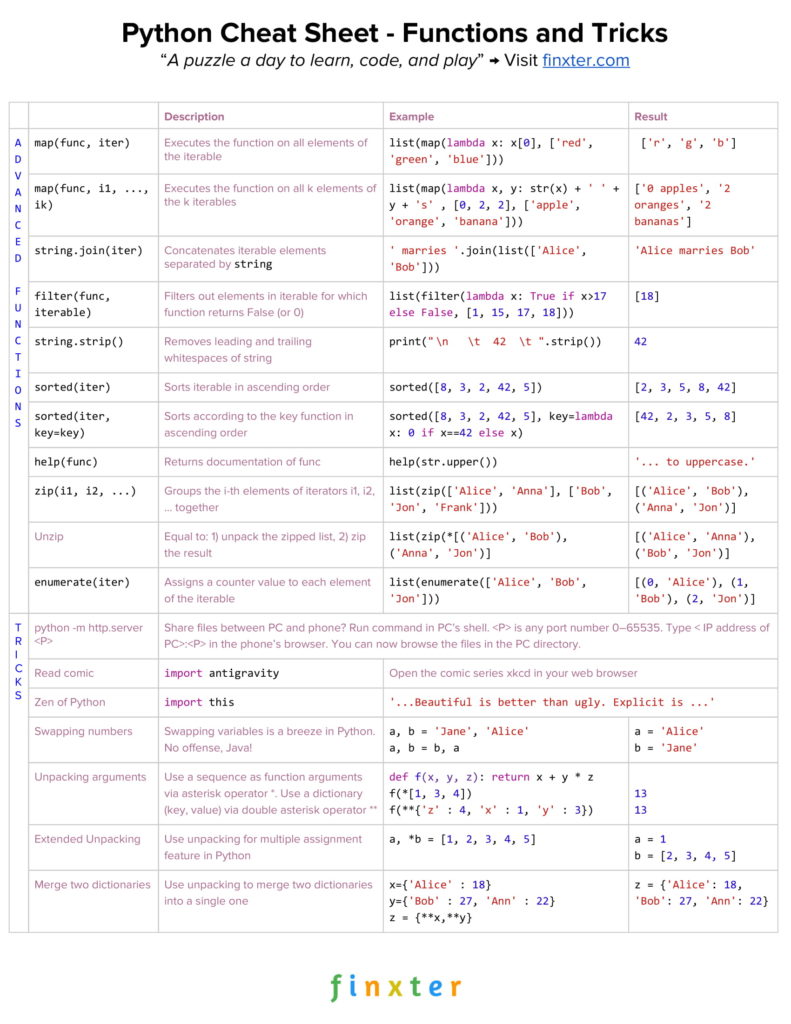
Want to learn Python well, but don’t have much time?
Then this course is for you. It contains 5 carefully designed PDF cheat sheets. Each cheat sheet takes you one step further into the rabbit hole.
You will learn practical Python concepts from the hand-picked examples and code snippets. The topics include basic keywords, simple and complex data types, crucial string and list methods, and powerful Python one-liners.
If you lead a busy life and do not want to compromise on quality, this is the cheat sheet course for you!
Dataquest Data Science Cheat Sheet – Python Basics
The wonderful team at Dataquest has put together this comprehensive beginner-level Python cheat sheet.
It covers all the basic data types, looping, and reading files. It’s beautifully designed and is the first of a series.
Dataquest Data Science Cheat Sheet – Intermediate
This intermediate-level cheat sheet is a follow-up of the other Dataquest cheat sheet. It contains intermediate dtype
methods, looping, and handling errors.
Dataquest NumPy
NumPy is at the heart of data science. Advanced libraries like scikit-learn, Tensorflow, Pandas, and Matplotlib are built on NumPy arrays.
You need to understand NumPy before you can thrive in data science and machine learning. The topics of this cheat sheet are creating arrays, combining arrays, scalar math, vector math, and statistics.
This is only one great NumPy cheat sheet—if you want to get more, check out our article on the 10 best NumPy cheat sheets!
Python For Data Science (Bokeh)
Want to master the visualization library Bokeh? This cheat sheet is for you! It contains all the basic Bokeh commands to get your beautiful visualizations going fast!
Pandas Cheat Sheet for Data Science
Pandas is everywhere. If you want to master “the Excel library for Python coders”, why not start with this cheat sheet? It’ll get you started fast and introduces the most important Pandas functions to you.
You can find a best-of article about the 7 best Pandas Cheat Sheets here.
Regular Expressions Cheat Sheet
Regex to the rescue! Regular expressions are wildly important for anyone who handles large amounts of text programmatically (ask Google).
This cheat sheet introduces the most important Regex commands for quick reference. Download & master these regular expressions!
If you love cheat sheets, here are some interesting references for you (lots of more PDF downloads):
Related Articles:
- [Collection] 11 Python Cheat Sheets Every Python Coder Must Own
- [Python OOP Cheat Sheet] A Simple Overview of Object-Oriented Programming
- [Collection] 15 Mind-Blowing Machine Learning Cheat Sheets to Pin to Your Toilet Wall
- Your 8+ Free Python Cheat Sheet [Course]
- Python Beginner Cheat Sheet: 19 Keywords Every Coder Must Know
- Python Functions and Tricks Cheat Sheet
- Python Cheat Sheet: 14 Interview Questions
- Beautiful Pandas Cheat Sheets
- 10 Best NumPy Cheat Sheets
- Python List Methods Cheat Sheet [Instant PDF Download]
- [Cheat Sheet] 6 Pillar Machine Learning Algorithms
Programming Humor – Python
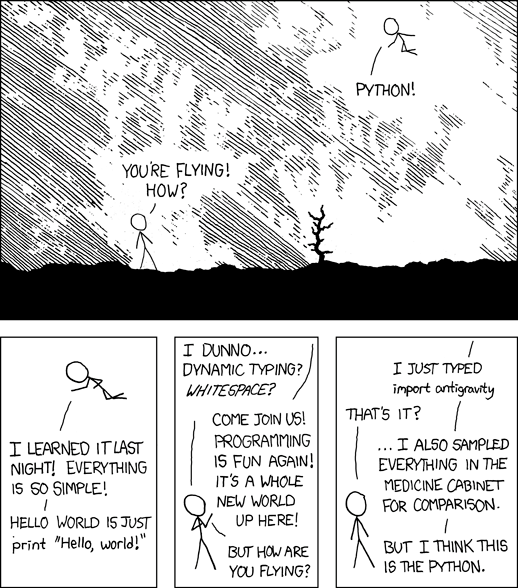
Where to Go From Here?
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
If your answer is YES!, consider becoming a Python freelance developer! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
September 26, 2024 at 10:32AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
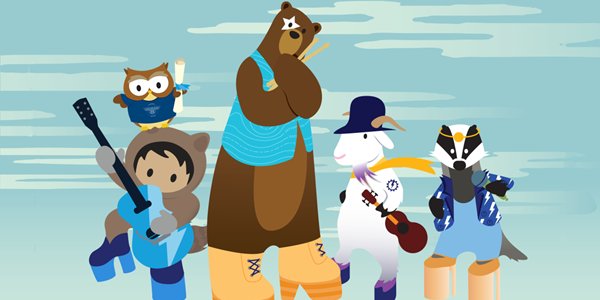
Post a Comment