Efficient String Concatenation in Python
by:
blow post content copied from Real Python
click here to view original post
Python string concatenation is a fundamental operation that combines multiple strings into a single string. In Python, you can concatenate strings using the +
operator or the +=
operator for appending. For more efficient concatenation of multiple strings, the .join()
method is recommended, especially when working with strings in a list. Other techniques include using StringIO
for large datasets or the print()
function for quick screen outputs.
By the end of this tutorial, you’ll understand that:
- You can concatenate strings in Python using the
+
operator and the+=
operator. - You can use
+=
to append a string to an existing string. - The
.join()
method is used to combine strings in a list in Python. - You can handle a stream of strings efficiently by using
StringIO
as a container with a file-like interface.
To get the most out of this tutorial, you should have a basic understanding of Python, especially its built-in string data type.
Get Your Code: Click here to download the free sample code that shows you how to efficiently concatenate strings in Python.
Doing String Concatenation With Python’s Plus Operator (+
)
String concatenation is a pretty common operation consisting of joining two or more strings together end to end to build a final string. Perhaps the quickest way to achieve concatenation is to take two separate strings and combine them with the plus operator (+
), which is known as the concatenation operator in this context:
>>> "Hello, " + "Pythonista!"
'Hello, Pythonista!'
>>> head = "String Concatenation "
>>> tail = "is Fun in Python!"
>>> head + tail
'String Concatenation is Fun in Python!'
Using the concatenation operator to join two strings provides a quick solution for concatenating only a few strings.
For a more realistic example, say you have an output line that will print an informative message based on specific criteria. The beginning of the message might always be the same. However, the end of the message will vary depending on different criteria. In this situation, you can take advantage of the concatenation operator:
>>> def age_group(age):
... if 0 <= age <= 9:
... result = "a Child!"
... elif 9 < age <= 18:
... result = "an Adolescent!"
... elif 19 < age <= 65:
... result = "an Adult!"
... else:
... result = "in your Golden Years!"
... print("You are " + result)
...
>>> age_group(29)
You are an Adult!
>>> age_group(14)
You are an Adolescent!
>>> age_group(68)
You are in your Golden Years!
In the above example, age_group()
prints a final message constructed with a common prefix and the string resulting from the conditional statement. In this type of use case, the plus operator is your best option for quick string concatenation in Python.
The concatenation operator has an augmented version that provides a shortcut for concatenating two strings together. The augmented concatenation operator (+=
) has the following syntax:
string += other_string
This expression will concatenate the content of string
with the content of other_string
. It’s equivalent to saying string = string + other_string
.
Here’s a short example of how the augmented concatenation operator works in practice:
>>> word = "Py"
>>> word += "tho"
>>> word += "nis"
>>> word += "ta"
>>> word
'Pythonista'
In this example, every augmented assignment adds a new syllable to the final word using the +=
operator. This concatenation technique can be useful when you have several strings in a list or any other iterable and want to concatenate them in a for
loop:
>>> def concatenate(iterable, sep=" "):
... sentence = iterable[0]
... for word in iterable[1:]:
... sentence += (sep + word)
... return sentence
...
>>> concatenate(["Hello,", "World!", "I", "am", "a", "Pythonista!"])
'Hello, World! I am a Pythonista!'
Inside the loop, you use the augmented concatenation operator to quickly concatenate several strings in a loop. Later you’ll learn about .join()
, which is an even better way to concatenate a list of strings.
Python’s concatenation operators can only concatenate string objects. If you use them with a different data type, then you get a TypeError
:
>>> "The result is: " + 42
Traceback (most recent call last):
...
TypeError: can only concatenate str (not "int") to str
>>> "Your favorite fruits are: " + ["apple", "grape"]
Traceback (most recent call last):
...
TypeError: can only concatenate str (not "list") to str
The concatenation operators don’t accept operands of different types. They only concatenate strings. A work-around to this issue is to explicitly use the built-in str()
function to convert the target object into its string representation before running the actual concatenation:
>>> "The result is: " + str(42)
'The result is: 42'
By calling str()
with your integer number as an argument, you’re retrieving the string representation of 42
, which you can then concatenate to the initial string because both are now string objects.
Read the full article at https://realpython.com/python-string-concatenation/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
November 24, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
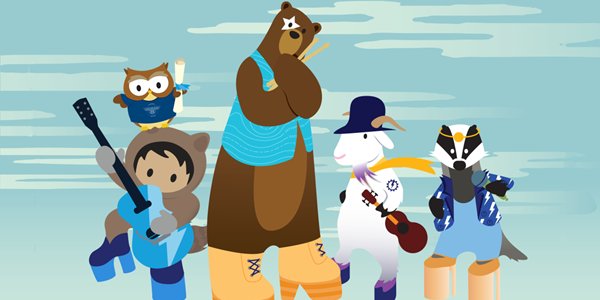
Post a Comment