Using the Python zip() Function for Parallel Iteration
by:
blow post content copied from Real Python
click here to view original post
Python’s zip()
function combines elements from multiple iterables. Calling zip()
generates an iterator that yields tuples, each containing elements from the input iterables. This function is essential for tasks like parallel iteration and dictionary creation, offering an efficient way to handle multiple sequences in Python programming.
By the end of this tutorial, you’ll understand that:
zip()
in Python aggregates elements from multiple iterables into tuples, facilitating parallel iteration.dict(zip())
creates dictionaries by pairing keys and values from two sequences.zip()
is lazy in Python, meaning it returns an iterator instead of a list.- There’s no
unzip()
function in Python, but the samezip()
function can reverse the process using the unpacking operator*
. - Alternatives to
zip()
includeitertools.zip_longest()
for handling iterables of unequal lengths.
In this tutorial, you’ll explore how to use zip()
for parallel iteration. You’ll also learn how to handle iterables of unequal lengths and discover the convenience of using zip()
with dictionaries. Whether you’re working with lists, tuples, or other data structures, understanding zip()
will enhance your coding skills and streamline your Python projects.
Free Bonus: 5 Thoughts On Python Mastery, a free course for Python developers that shows you the roadmap and the mindset you’ll need to take your Python skills to the next level.
Understanding the Python zip()
Function
zip()
is available in the built-in namespace. If you use dir()
to inspect __builtins__
, then you’ll see zip()
at the end of the list:
>>> dir(__builtins__)
['ArithmeticError', 'AssertionError', 'AttributeError', ..., 'zip']
You can see that 'zip'
is the last entry in the list of available objects.
According to the official documentation, Python’s zip()
function behaves as follows:
Returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the argument sequences or iterables. The iterator stops when the shortest input iterable is exhausted. With a single iterable argument, it returns an iterator of 1-tuples. With no arguments, it returns an empty iterator. (Source)
You’ll unpack this definition throughout the rest of the tutorial. As you work through the code examples, you’ll see that Python zip operations work just like the physical zipper on a bag or pair of jeans. Interlocking pairs of teeth on both sides of the zipper are pulled together to close an opening. In fact, this visual analogy is perfect for understanding zip()
, since the function was named after physical zippers!
Using zip()
in Python
The signature of Python’s zip()
function is zip(*iterables, strict=False)
. You’ll learn more about strict
later. The function takes in iterables as arguments and returns an iterator. This iterator generates a series of tuples containing elements from each iterable. zip()
can accept any type of iterable, such as files, lists, tuples, dictionaries, sets, and so on.
Passing n
Arguments
If you use zip()
with n
arguments, then the function will return an iterator that generates tuples of length n
. To see this in action, take a look at the following code block:
>>> numbers = [1, 2, 3]
>>> letters = ["a", "b", "c"]
>>> zipped = zip(numbers, letters)
>>> zipped # Holds an iterator object
<zip object at 0x7fa4831153c8>
>>> type(zipped)
<class 'zip'>
>>> list(zipped)
[(1, 'a'), (2, 'b'), (3, 'c')]
Here, you use zip(numbers, letters)
to create an iterator that produces tuples of the form (x, y)
. In this case, the x
values are taken from numbers
and the y
values are taken from letters
. Notice how the Python zip()
function returns an iterator. To retrieve the final list object, you need to use list()
to consume the iterator.
If you’re working with sequences like lists, tuples, or strings, then your iterables are guaranteed to be evaluated from left to right. This means that the resulting list of tuples will take the form [(numbers[0], letters[0]), (numbers[1], letters[1]),..., (numbers[n], letters[n])]
. However, for other types of iterables (like sets), you might see some weird results:
>>> s1 = {2, 3, 1}
>>> s2 = {"b", "a", "c"}
>>> list(zip(s1, s2))
[(1, 'a'), (2, 'c'), (3, 'b')]
In this example, s1
and s2
are set
objects, which don’t keep their elements in any particular order. This means that the tuples returned by zip()
will have elements that are paired up randomly. If you’re going to use the Python zip()
function with unordered iterables like sets, then this is something to keep in mind.
Passing No Arguments
You can call zip()
with no arguments as well. In this case, you’ll simply get an empty iterator:
>>> zipped = zip()
>>> zipped
<zip object at 0x7f196294a488>
>>> list(zipped)
[]
Here, you call zip()
with no arguments, so your zipped
variable holds an empty iterator. If you consume the iterator with list()
, then you’ll see an empty list as well.
Read the full article at https://realpython.com/python-zip-function/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
November 17, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
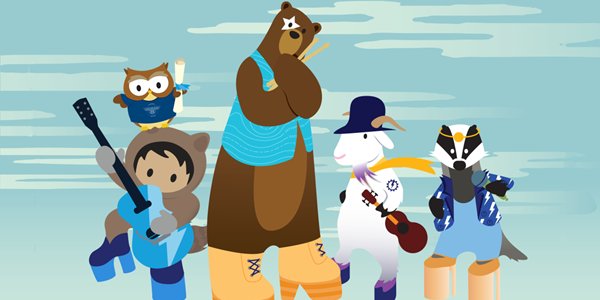
Post a Comment