Basic Input and Output in Python
by:
blow post content copied from Real Python
click here to view original post
For a program to be useful, it often needs to communicate with the outside world. In Python, the input()
function allows you to capture user input from the keyboard, while you can use the print()
function to display output to the console.
These built-in functions allow for basic user interaction in Python scripts, enabling you to gather data and provide feedback. If you want to go beyond the basics, then you can even use them to develop applications that are not only functional but also user-friendly and responsive.
By the end of this tutorial, you’ll know how to:
- Take user input from the keyboard with
input()
- Display output to the console with
print()
- Use
readline
to improve the user experience when collecting input on UNIX-like systems - Format output using the
sep
andend
keyword arguments ofprint()
To get the most out of this tutorial, you should have a basic understanding of Python syntax and familiarity with using the Python interpreter and running Python scripts.
Get Your Code: Click here to download the free sample code that you’ll use to learn about basic input and output in Python.
Take the Quiz: Test your knowledge with our interactive “Basic Input and Output in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Basic Input and Output in PythonIn this quiz, you'll test your understanding of Python's built-in functions for user interaction, namely input() and print(). These functions allow you to capture user input from the keyboard and display output to the console, respectively.
Reading Input From the Keyboard
Programs often need to obtain data from users, typically through keyboard input. In Python, one way to collect user input from the keyboard is by calling the input()
function:
The input()
function pauses program execution to allow you to type in a line of input from the keyboard. Once you press the Enter key, all characters typed are read and returned as a string, excluding the newline character generated by pressing Enter.
If you add text in between the parentheses, effectively passing a value to the optional prompt
argument, then input()
displays the text you entered as a prompt:
>>> name = input("Please enter your name: ")
Please enter your name: John Doe
>>> name
'John Doe'
Adding a meaningful prompt will assist your user in understanding what they’re supposed to input, which makes for a better user experience.
The input()
function always reads the user’s input as a string. Even if you type characters that resemble numbers, Python will still treat them as a string:
1>>> number = input("Enter a number: ")
2Enter a number: 50
3
4>>> type(number)
5<class 'str'>
6
7>>> number + 100
8Traceback (most recent call last):
9 File "<python-input-1>", line 1, in <module>
10 number + 100
11 ~~~~~~~^~~~~
12TypeError: can only concatenate str (not "int") to str
In the example above, you wanted to add 100
to the number entered by the user. However, the expression number + 100
on line 7 doesn’t work because number
is a string ("50"
) and 100
is an integer. In Python, you can’t combine a string and an integer using the plus (+
) operator.
You wanted to perform a mathematical operation using two integers, but because input()
always returns a string, you need a way to read user input as a numeric type. So, you’ll need to convert the string to the appropriate type:
>>> number = int(input("Enter a number: "))
Enter a number: 50
>>> type(number)
<class 'int'>
>>> number + 100
150
In this updated code snippet, you use int()
to convert the user input to an integer right after collecting it. Then, you assign the converted value to the name number
. That way, the calculation number + 100
has two integers to add. The calculation succeeds and Python returns the correct sum.
Note: When you convert user input to a numeric type using functions like int()
in a real-world scenario, it’s crucial to handle potential exceptions to prevent your program from crashing due to invalid input.
The input()
function lets you collect information from your users. But once your program has calculated a result, how do you display it back to them? Up to this point, you’ve seen results displayed automatically as output in the interactive Python interpreter session.
However, if you ran the same code from a file instead, then Python would still calculate the values, but you wouldn’t see the results. To display output in the console, you can use Python’s print()
function, which lets you show text and data to your users.
Writing Output to the Console
In addition to obtaining data from the user, a program will often need to present data back to the user. In Python, you can display data to the console with the print()
function.
Read the full article at https://realpython.com/python-input-output/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 02, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
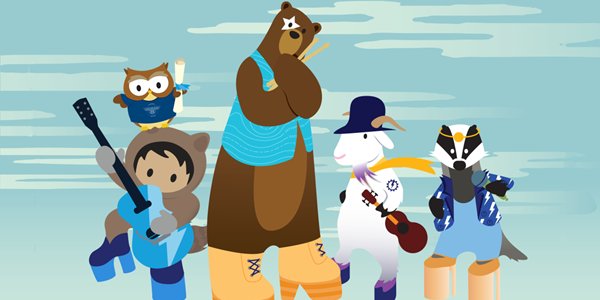
Post a Comment