Dictionaries in Python :
by:
blow post content copied from Real Python
click here to view original post
Python dictionaries are a powerful built-in data type that allows you to store key-value pairs for efficient data retrieval and manipulation. Learning about them is essential for developers who want to process data efficiently. In this tutorial, you’ll explore how to create dictionaries using literals and the dict()
constructor, as well as how to use Python’s operators and built-in functions to manipulate them.
By learning about Python dictionaries, you’ll be able to access values through key lookups and modify dictionary content using various methods. This knowledge will help you in data processing, configuration management, and dealing with JSON and CSV data.
By the end of this tutorial, you’ll understand that:
- A dictionary in Python is a mutable collection of key-value pairs that allows for efficient data retrieval using unique keys.
- Both
dict()
and{}
can create dictionaries in Python. Use{}
for concise syntax anddict()
for dynamic creation from iterable objects. dict()
is a class used to create dictionaries. However, it’s commonly called a built-in function in Python..__dict__
is a special attribute in Python that holds an object’s writable attributes in a dictionary.- Python
dict
is implemented as a hashmap, which allows for fast key lookups.
To get the most out of this tutorial, you should be familiar with basic Python syntax and concepts such as variables, loops, and built-in functions. Some experience with basic Python data types will also be helpful.
Get Your Code: Click here to download the free sample code that you’ll use to learn about dictionaries in Python.
Take the Quiz: Test your knowledge with our interactive “Python Dictionaries” quiz. You’ll receive a score upon completion to help you track your learning progress:
Getting Started With Python Dictionaries
Dictionaries are one of Python’s most important and useful built-in data types. They provide a mutable collection of key-value pairs that lets you efficiently access and mutate values through their corresponding keys:
>>> config = {
... "color": "green",
... "width": 42,
... "height": 100,
... "font": "Courier",
... }
>>> # Access a value through its key
>>> config["color"]
'green'
>>> # Update a value
>>> config["font"] = "Helvetica"
>>> config
{
'color': 'green',
'width': 42,
'height': 100,
'font': 'Helvetica'
}
A Python dictionary consists of a collection of key-value pairs, where each key corresponds to its associated value. In this example, "color"
is a key, and "green"
is the associated value.
Dictionaries are a fundamental part of Python. You’ll find them behind core concepts like scopes and namespaces as seen with the built-in functions globals()
and locals()
:
>>> globals()
{
'__name__': '__main__',
'__doc__': None,
'__package__': None,
...
}
The globals()
function returns a dictionary containing key-value pairs that map names to objects that live in your current global scope.
Python also uses dictionaries to support the internal implementation of classes. Consider the following demo class:
>>> class Number:
... def __init__(self, value):
... self.value = value
...
>>> Number(42).__dict__
{'value': 42}
The .__dict__
special attribute is a dictionary that maps attribute names to their corresponding values in Python classes and objects. This implementation makes attribute and method lookup fast and efficient in object-oriented code.
You can use dictionaries to approach many programming tasks in your Python code. They come in handy when processing CSV and JSON files, working with databases, loading configuration files, and more.
Python’s dictionaries have the following characteristics:
- Mutable: The dictionary values can be updated in place.
- Dynamic: Dictionaries can grow and shrink as needed.
- Efficient: They’re implemented as hash tables, which allows for fast key lookup.
- Ordered: Starting with Python 3.7, dictionaries keep their items in the same order they were inserted.
The keys of a dictionary have a couple of restrictions. They need to be:
- Hashable: This means that you can’t use unhashable objects like lists as dictionary keys.
- Unique: This means that your dictionaries won’t have duplicate keys.
In contrast, the values in a dictionary aren’t restricted. They can be of any Python type, including other dictionaries, which makes it possible to have nested dictionaries.
It’s important to note that dictionaries are collections of pairs. So, you can’t insert a key without its corresponding value or vice versa. Since they come as a pair, you always have to insert a key with its corresponding value.
Note: In some situations, you may want to add keys to a dictionary without deciding what the associated value should be. In those cases, you can use the .setdefault()
method to create keys with a default or placeholder value.
Read the full article at https://realpython.com/python-dicts/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 16, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
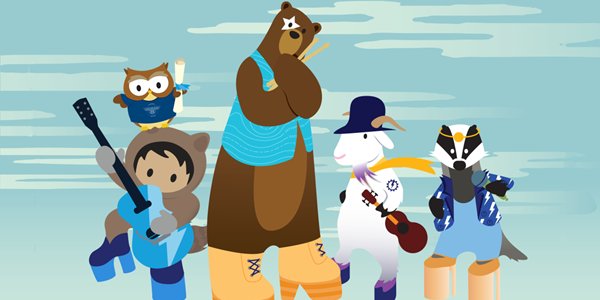
Post a Comment