Python Timer Functions: Three Ways to Monitor Your Code
by:
blow post content copied from Real Python
click here to view original post
A timer is a powerful tool for monitoring the performance of your Python code. By using the time.perf_counter()
function, you can measure execution time with exceptional precision, making it ideal for benchmarking. Using a timer involves recording timestamps before and after a specific code block and calculating the time difference to determine how long your code took to run.
In this tutorial, you’ll explore three different approaches to implementing timers: classes, decorators, and context managers. Each method offers unique advantages, and you’ll learn when and how to use them to achieve optimal results. Plus, you’ll have a fully functional Python timer that can be applied to any program to measure execution time efficiently.
By the end of this tutorial, you’ll understand that:
time.perf_counter()
is the best choice for accurate timing in Python due to its high resolution.- You can create custom timer classes to encapsulate timing logic and reuse it across multiple parts of your program.
- Using decorators lets you seamlessly add timing functionality to existing functions without altering their code.
- You can leverage context managers to neatly measure execution time in specific code blocks, improving both resource management and code clarity.
Along the way, you’ll gain deeper insights into how classes, decorators, and context managers work in Python. As you explore real-world examples, you’ll discover how these concepts can not only help you measure code performance but also enhance your overall Python programming skills.
Decorators Q&A Transcript: Click here to get access to a 25-page chat log from our Python decorators Q&A session in the Real Python Community Slack where we discussed common decorator questions.
Python Timers
First, you’ll take a look at some example code that you’ll use throughout the tutorial. Later, you’ll add a Python timer to this code to monitor its performance. You’ll also learn some of the simplest ways to measure the running time of this example.
Python Timer Functions
If you check out the built-in time
module in Python, then you’ll notice several functions that can measure time:
Python 3.7 introduced several new functions, like thread_time()
, as well as nanosecond versions of all the functions above, named with an _ns
suffix. For example, perf_counter_ns()
is the nanosecond version of perf_counter()
. You’ll learn more about these functions later. For now, note what the documentation has to say about perf_counter()
:
Return the value (in fractional seconds) of a performance counter, i.e. a clock with the highest available resolution to measure a short duration. (Source)
First, you’ll use perf_counter()
to create a Python timer. Later, you’ll compare this with other Python timer functions and learn why perf_counter()
is usually the best choice.
Example: Download Tutorials
To better compare the different ways that you can add a Python timer to your code, you’ll apply different Python timer functions to the same code example throughout this tutorial. If you already have code that you’d like to measure, then feel free to follow the examples with that instead.
The example that you’ll use in this tutorial is a short function that uses the realpython-reader
package to download the latest tutorials available here on Real Python. To learn more about the Real Python Reader and how it works, check out How to Publish an Open-Source Python Package to PyPI. You can install realpython-reader
on your system with pip
:
$ python -m pip install realpython-reader
Then, you can import the package as reader
.
You’ll store the example in a file named latest_tutorial.py
. The code consists of one function that downloads and prints the latest tutorial from Real Python:
latest_tutorial.py
1from reader import feed
2
3def main():
4 """Download and print the latest tutorial from Real Python"""
5 tutorial = feed.get_article(0)
6 print(tutorial)
7
8if __name__ == "__main__":
9 main()
realpython-reader
handles most of the hard work:
- Line 1 imports
feed
fromrealpython-reader
. This module contains functionality for downloading tutorials from the Real Python feed. - Line 5 downloads the latest tutorial from Real Python. The number
0
is an offset, where0
means the most recent tutorial,1
is the previous tutorial, and so on. - Line 7 prints the tutorial to the console.
- Line 9 calls
main()
when you run the script.
When you run this example, your output will typically look something like this:
$ python latest_tutorial.py
# Python Timer Functions: Three Ways to Monitor Your Code
A timer is a powerful tool for monitoring the performance of your Python
code. By using the `time.perf_counter()` function, you can measure execution
time with exceptional precision, making it ideal for benchmarking. Using a
timer involves recording timestamps before and after a specific code block and
calculating the time difference to determine how long your code took to run.
[ ... ]
## Read the full article at https://realpython.com/python-timer/ »
* * *
Read the full article at https://realpython.com/python-timer/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 08, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
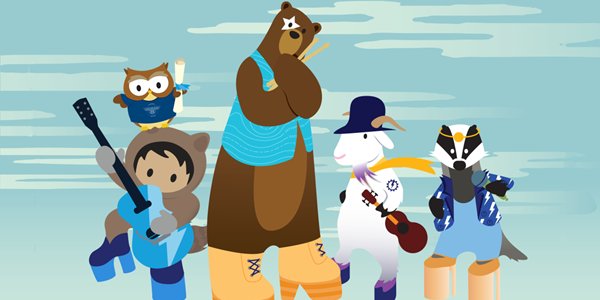
Post a Comment