Using and Creating Global Variables in Your Python Functions
by:
blow post content copied from Real Python
click here to view original post
In Python, global variables are accessible across your entire program, including within functions. Understanding how Python handles global variables is key to writing efficient code. This tutorial will guide you through accessing and modifying global variables in Python functions using the global
keyword and the globals()
function. You’ll also learn to manage scope and avoid potential conflicts between local and global variables.
You’ll explore how to create global variables inside functions and apply strategies to minimize their use, ensuring your code remains clean and maintainable. After reading this tutorial, you’ll be adept at managing global variables and understanding their impact on your Python code.
By the end of this tutorial, you’ll understand that:
- A global variable in Python is a variable defined at the module level, accessible throughout the program.
- Accessing and modifying global variables inside Python functions can be achieved using the
global
keyword or theglobals()
function. - Python handles name conflicts by searching scopes from local to built-in, potentially causing name shadowing challenges.
- Creating global variables inside a function is possible using the
global
keyword orglobals()
, but it’s generally not recommended. - Strategies to avoid global variables include using constants, passing arguments, and employing classes and methods to encapsulate state.
To follow along with this tutorial, you should have a solid understanding of Python programming, including fundamental concepts such as variables, data types, scope, mutability, functions, and classes.
Get Your Code: Click here to download the free sample code that you’ll use to understand when and how to work with global variables in your Python functions.
Take the Quiz: Test your knowledge with our interactive “Using and Creating Global Variables in Your Python Functions” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Using and Creating Global Variables in Your Python FunctionsIn this quiz, you'll test your understanding of how to use global variables in Python functions. With this knowledge, you'll be able to share data across an entire program, modify and create global variables within functions, and understand when to avoid using global variables.
Using Global Variables in Python Functions
Global variables are those that you can access and modify from anywhere in your code. In Python, you’ll typically define global variables at the module level. So, the containing module is their scope.
Note: You can also define global variables inside functions, as you’ll learn in the section Creating Global Variables Inside a Function.
Once you’ve defined a global variable, you can use it from within the module itself or from within other modules in your code. You can also use global variables in your functions. However, those cases can get a bit confusing because of differences between accessing and modifying global variables in functions.
To understand these differences, consider that Python can look for variables in four different scopes:
- The local, or function-level, scope, which exists inside functions
- The enclosing, or non-local, scope, which appears in nested functions
- The global scope, which exists at the module level
- The built-in scope, which is a special scope for Python’s built-in names
To illustrate, say that you’re inside an inner function. In that case, Python can look for names in all four scopes.
When you access a variable in that inner function, Python first looks inside that function. If the variable doesn’t exist there, then Python continues with the enclosing scope of the outer function. If the variable isn’t defined there either, then Python moves to the global and built-in scopes in that order. If Python finds the variable, then you get the value back. Otherwise, you get a NameError
:
>>> # Global scope
>>> def outer_func():
... # Non-local scope
... def inner_func():
... # Local scope
... print(some_variable)
... inner_func()
...
>>> outer_func()
Traceback (most recent call last):
...
NameError: name 'some_variable' is not defined
>>> some_variable = "Hello from global scope!"
>>> outer_func()
Hello from global scope!
When you launch an interactive session, it starts off at the module level of global scope. In this example, you have outer_func()
, which defines inner_func()
as a nested function. From the perspective of this nested function, its own code block represents the local scope, while the outer_func()
code block before the call to inner_func()
represents the non-local scope.
If you call outer_func()
without defining some_variable
in either of your current scopes, then you get a NameError
exception because the name isn’t defined.
If you define some_variable
in the global scope and then call outer_func()
, then you get Hello!
on your screen. Internally, Python has searched the local, non-local, and global scopes to find some_variable
and print its content. Note that you can define this variable in any of the three scopes, and Python will find it.
This search mechanism makes it possible to use global variables from inside functions. However, while taking advantage of this feature, you can face a few issues. For example, accessing a variable works, but directly modifying a variable doesn’t work:
>>> number = 42
>>> def access_number():
... return number
...
>>> access_number()
42
>>> def modify_number():
... number = 7
...
>>> modify_number()
>>> number
42
The access_number()
function works fine. It looks for number
and finds it in the global scope. In contrast, modify_number()
doesn’t work as expected. Why doesn’t this function update the value of your global variable, number
? The problem is the scope of the variable. You can’t directly modify a variable from a high-level scope like global in a lower-level scope like local.
Internally, Python assumes that any name directly assigned within a function is local to that function. Therefore, the local name, number
, shadows its global sibling.
In this sense, global variables behave as read-only names. You can access their values, but you can’t modify them.
Note: The discussion about modifying global variables inside functions revolves around assignment operations rather than in-place mutations of mutable objects. You’ll learn about the effects of mutability on global variables in the section Understanding How Mutability Affects Global Variables.
Read the full article at https://realpython.com/python-use-global-variable-in-function/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 08, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
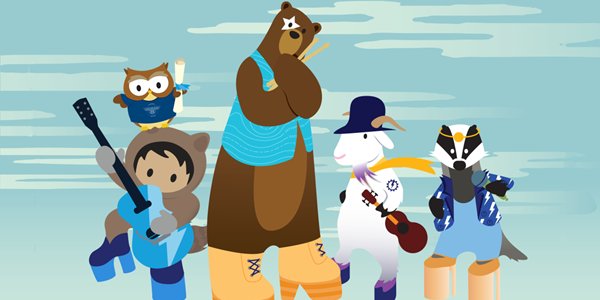
Post a Comment