Getters and Setters: Manage Attributes in Python :
by:
blow post content copied from Real Python
click here to view original post
Getter and setter methods allow you to access and mutate non-public attributes while maintaining encapsulation. In Python, you’ll typically expose attributes as part of your public API and use properties when you need attributes with functional behavior. This tutorial guides you through writing getter and setter methods, replacing them with properties, and exploring alternatives like descriptors for optimized attribute management.
By the end of this tutorial, you’ll understand that:
- Getter and setter methods allow you to access and modify data attributes while maintaining encapsulation.
- Python properties can replace getters and setters, providing a more Pythonic way to manage attributes with functional behavior.
- You typically avoid using getters and setters in Python unless necessary, as properties and descriptors offer more flexible solutions.
- Descriptors are an advanced Python feature that enable reusable attributes with attached behaviors across different classes.
- In some scenarios, inheritance limitations may make traditional getters and setters preferable over properties.
Even though properties are the Pythonic way to go, they can have some practical drawbacks. Because of this, you’ll find some situations where getters and setters are preferable over properties. This tutorial will delve into the nuances of using different approaches, equipping you with the knowledge to make informed decisions about attribute management in your classes.
To get the most out of this tutorial, you should be familiar with Python object-oriented programming. It’ll also be a plus if you have basic knowledge of Python properties and descriptors.
Source Code: Click here to get the free source code that shows you how and when to use getters, setters, and properties in Python.
Take the Quiz: Test your knowledge with our interactive “Getters and Setters: Manage Attributes in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Getters and Setters: Manage Attributes in PythonIn this quiz, you'll test your understanding of Python's getter and setter methods, as well as properties. You'll learn when to use these tools and how they can help maintain encapsulation in your classes.
Getting to Know Getter and Setter Methods
When you define a class in object-oriented programming (OOP), you’ll likely end up with some instance and class attributes. These attributes are just variables that you can access through the instance, the class, or both.
Attributes hold the internal state of objects. In many cases, you’ll need to access and mutate this state, which involves accessing and mutating the attributes. Typically, you’ll have at least two ways to access and mutate attributes. You can either:
- Access and mutate the attribute directly
- Use methods to access and mutate the attribute
If you expose the attributes of a class to your users, then those attributes automatically become part of the class’s public API. They’ll be public attributes, which means that your users will directly access and mutate the attributes in their code.
Having an attribute that’s part of a class’s API will become a problem if you need to change the internal implementation of the attribute itself. A clear example of this issue is when you want to turn a stored attribute into a computed one. A stored attribute will immediately respond to access and mutation operations by just retrieving and storing data, while a computed attribute will run computations before such operations.
The problem with regular attributes is that they can’t have an internal implementation because they’re just variables. So, changing an attribute’s internal implementation will require converting the attribute into a method, which will probably break your users’ code. Why? Because they’ll have to change attribute access and mutation operations into method calls throughout their codebase if they want the code to continue working.
To deal with this kind of issue, some programming languages, like Java and C++, require you to provide methods for manipulating the attributes of your classes. These methods are commonly known as getter and setter methods. You can also find them referred to as accessor and mutator methods.
What Are Getter and Setter Methods?
Getter and setter methods are quite popular in many object-oriented programming languages. So, it’s pretty likely that you’ve heard about them already. As a rough definition, you can say that getters and setters are:
- Getter: A method that allows you to access an attribute in a given class
- Setter: A method that allows you to set or mutate the value of an attribute in a class
In OOP, the getter and setter pattern suggests that public attributes should be used only when you’re sure that no one will ever need to attach behavior to them. If an attribute is likely to change its internal implementation, then you should use getter and setter methods.
Implementing the getter and setter pattern requires:
- Making your attributes non-public
- Writing getter and setter methods for each attribute
For example, say that you need to write a Label
class with text and font attributes. If you were to use getter and setter methods to manage these attributes, then you’d write the class like in the following code:
label.py
class Label:
def __init__(self, text, font):
self._text = text
self._font = font
def get_text(self):
return self._text
def set_text(self, value):
self._text = value
def get_font(self):
return self._font
def set_font(self, value):
self._font = value
In this example, the constructor of Label
takes two arguments, text
and font
. These arguments are stored in the ._text
and ._font
non-public instance attributes, respectively.
Then you define getter and setter methods for both attributes. Typically, getter methods return the target attribute’s value, while setter methods take a new value and assign it to the underlying attribute.
Read the full article at https://realpython.com/python-getter-setter/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
January 20, 2025 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
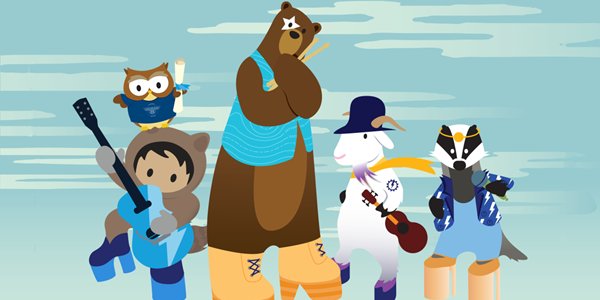
Post a Comment